The Problem: Missing Placeholders
![]() |
Figure 1 - Placeholder text in the Settings App. |
Solving the Problem
- Create an extension that adds a calculated property named placeholder to the UITextView class
- In the extension, display the label when there is no text in the text view, and hide it when there is text.
Solution 1: UITextViewDelegate
Solution 2: NSNotificationCenter
Creating a Notification Proxy
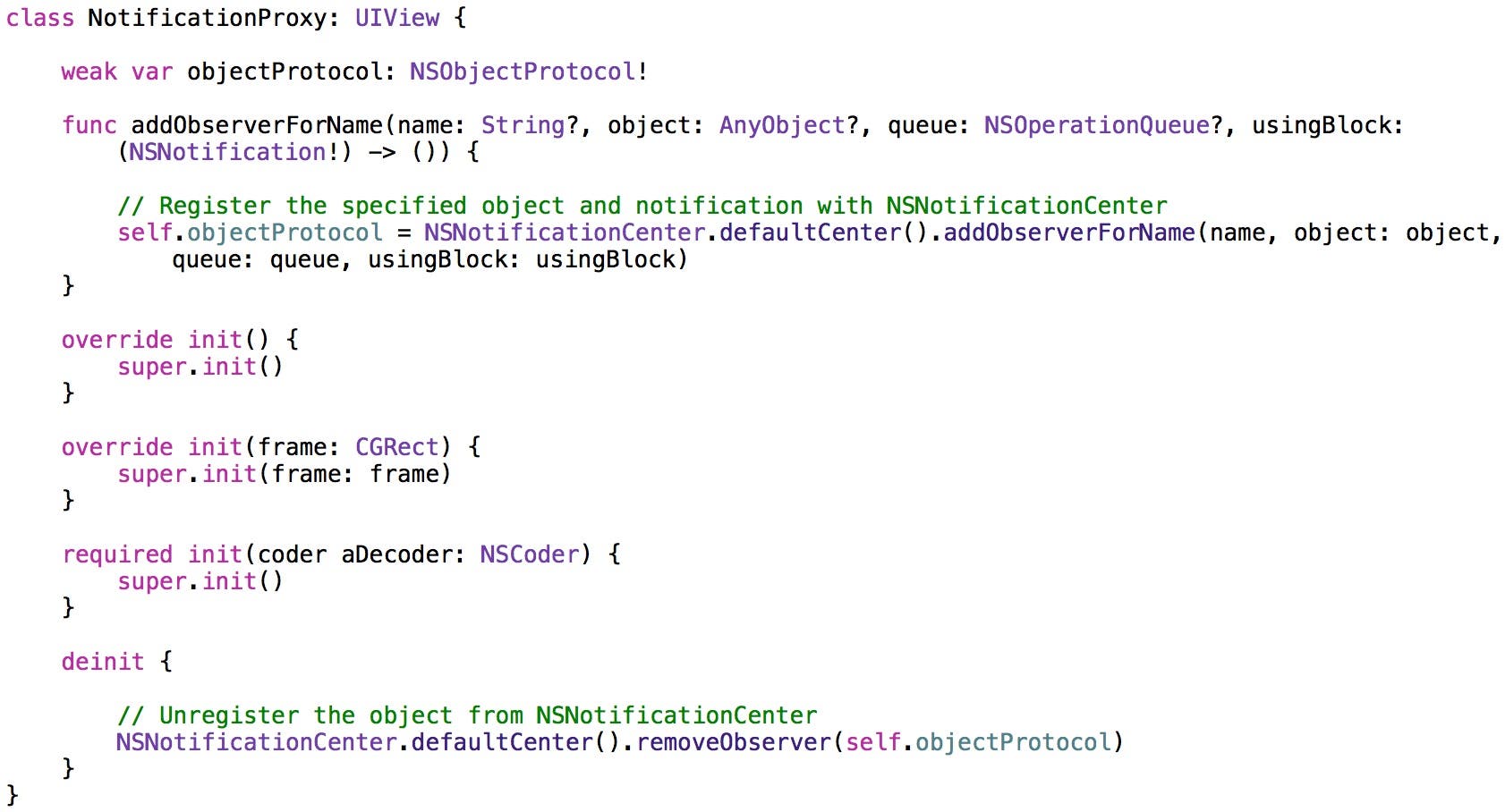
![]() |
Figure 2 - The main scene contains a text view |
The Notification Proxy at Run Time
![]() |
Figure 3 - The order of messages passed between all objects at run time |
- When the UITextView placeholder text is set at run time it fires the extension’s placeholder computed property’s code.
- The UITextView extensions’s addPlaceholderLabel method is called.
- The addPlaceholderLabel method creates a placeholder label and sets its text.
- The placeholder label is added to the UITextView.
- A Notification Proxy object is created.
- The UITextView extension calls the Notification Proxy’s addObserverForName:withBlock: method, specifying UITextViewTextDidChangeNotification as the name of the notification it wants to observe. It also passes a block of code to be executed when the notification occurs.
- The Notification Proxy object registers the UITextView with NSNotificationCenter, also passing through the notification event to be observed and the UITextView block to be executed when the notification occurs.
- The UITextView object adds the Notification Proxy to itself as a subview.
- When a UITextViewTextDidChangeNotification occurs, NSNotificationCenter calls the block on the UITextView extension.
- The UITextView extension sets the placeholder label’s hidden property to true or false, depending on whether or not there is any text entered in the text view.
- When the UITextView is deallocated at run time, the placeholder label and notification proxy object are deallocated.
- When the Notification Proxy object is deallocated, it unregisters the UITextView from NSNotificationCenter.
Let’s Take it for a Test Run!
- In Xcode’s Scheme control, select one of the simulators such as iPhone 6.
- Click Xcode’s Run button.
- When the app appears in the Simulator, you can see the placeholder text (Figure 4).
![]() |
Figure 4 - The placeholder text at run time |
![]() |
Figure 5 - The placeholder disappears |
- If you delete all the characters you typed, the placeholder reappears.